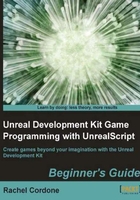
Time for action – Concatenation
The good news is we've been using concatenation for awhile now, in our log lines. The two operators are the at symbol (@
) and the dollar sign ($
). The only difference between the two is whether or not we want a space in between the strings we're joining.
- Let's write some code.
var string String1, String2, AtSign, DollarSign; function PostBeginPlay() { AtSign = String1 @ String2; DollarSign = String1 $ String2; 'log("At sign:" @ AtSign); 'log("Dollar sign:" @ DollarSign); } defaultproperties { String1="This is" String2="a test." }
Looking at the log shows us the minor difference between the two:
[0007.77] ScriptLog: At sign: This is a test. [0007.77] ScriptLog: Dollar sign: This isa test.
The choice between them is as simple as the space between the joined strings.
- The concatenation operators can also be used with equal signs to shorten the code and get rid of the need for extra variables. The
@
code could also be written like this:var string String1, String2; function PostBeginPlay() { String1 @= String2; 'log("String1:" @ String1); } defaultproperties { String1="This is" String2="a test." }
The log ends up the same:
[0007.56] ScriptLog: String1: This is a test.
What just happened?
Concatenation is specific to strings and easy to remember. There are instances when you'd want to use the dollar sign and ones where the @
symbol is needed, it just depends on what we're trying to do with the string. As an example, death messages often use the @
symbol to join the player name to the death message so there is a space in between them, while strings that tell the game which level to load use the dollar sign specifically to avoid spaces.
Variable functions
There are many other variable functions we can use; some of them are handy to know. Let's go over a few.
Rand(int Max)
: Returns a random int between 0 and the maximum number specified. Note that this will never return Max itself. As an example,Rand(10)
would return a random number 0-9.Min(int A, int B)
: Returns the smaller of the two ints.Min(5, 2)
would return 2.Max(int A, int B)
: Returns the higher of the two numbers.Max(3, 8)
would return 8.Clamp(int V, int A, int B)
: Clamps V to be between A and B. If V is lesser than A it would return A, if it were greater than B it would return B. If it were already in between, V would stay the same.Clamp(3, 5, 8)
would return 5 whileClamp(5, 2, 9)
would return 5.
FRand()
: The same as Rand, but returns a random float between 0.0 and 1.0.FMin(float A, float B)
: The float version of Min.FMax(float A, float B)
: The float version of Max.FClamp(float V, float A, float B)
: The float version of Clamp. Floats and ints can be used in either version of these three depending on whether or not we want the result to be truncated.
Len(string S)
: Returns the length of the string as an int. This includes spaces and any symbols.InStr(string S, string T)
: Returns the position of string T in string S, or -1 if T can't be found in S. This is useful for figuring out if a string has a certain word in it, since we can check if the result is >= 0 to indicate that the word is there.Left(string S, int I)
: Returns the left I number of characters.Left("Something", 3)
would return "Som".Right(string S, int I)
: Returns the right I number of characters.Right("Something", 3)
would return "ing".Mid(string S, int I, optional int J)
: Returns a string from position I.Mid("Something", 2, 3)
returns "met". If J isn't specified this has the same effect as Right.Caps(string S)
: Returns the string in all caps.Locs(string S)
: Returns the string in all lowercase.Repl(string S, string Match, string With, optional bool bCaseSensitive)
: Replaces Match with With in string S.Repl("Something", "Some", "No")
would return "Nothing". The optional bool specifies if you only want it to replace With if letters have the same case as Match.