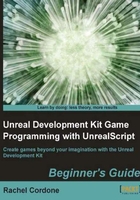
Time for action – Something
- As an example of what NOT to do, let's take this code.
var int Int1; function PostBeginPlay() { Int1 = 5; While(Int1 > 0) { Int1 = 5; } }
- When we run the game with this code, it will crash.
It is EXTREMELY IMPORTANT to always make sure the "while" condition will be met to avoid infinite loop crashes.
- Let's take a look at the right way to use it:
var int Int1; function PostBeginPlay() { While(Int1 < 5) { 'log("Int1" @ Int1); Int1++; } }
In this case,
Int1
will keep incrementing until it reaches 5, and the While loop will exit. - We could also use a statement called
break
to exit the loop:var int Int1; function PostBeginPlay() { While(Int1 < 5) { 'log("Int1" @ Int1); Int1++; if(Int1 == 3) break; } }
In this case, the loop will exit when
Int1
reaches 3 instead of continuing until it hits 5. - Another statement we can use is called continue. Instead of exiting the loop completely, it just skips to the next cycle.
var int Int1; function PostBeginPlay() { While(Int1 < 5) { 'log("Int1" @ Int1); Int1++; if(Int1 == 3) continue; 'log("This will not log if Int1 is 3"); } }
In this case, the loop will keep going until Int1
hits 5, but when it's equal to 3 the continue statement will cause it to skip the rest of the code for that loop and move on to the next loop.
What just happened?
Using while statements can be handy when you don't know the number of loops you'll need beforehand, or if it would change during play. You always have to make sure the loop will be able to finish though; crashing the game is a very real concern when using while loops.