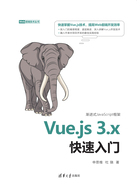
上QQ阅读APP看书,第一时间看更新
2.2.4 JS代码部分
注释如下所示。
<script type="text/javascript"> window.onload=function(){ // 下面的代码,表示使用了浏览器的 localStorage进行存储。同时定义了fetch()和save() // 用来方便操作 var STORAGE_KEY = 'todos-vuejs' var todoStorage = { fetch: function () { var todos = JSON.parse(localStorage.getItem(STORAGE_KEY) || '[]') todos.forEach(function (todo, index) { todo.id = index }) todoStorage.uid = todos.length return todos }, save: function (todos) { localStorage.setItem(STORAGE_KEY, JSON.stringify(todos)) } } // 定义过滤器,可以分别对 ative、completed和 all进行过滤 var filters = { all: function (todos) { return todos }, active: function (todos) { return todos.filter(function (todo) { return !todo.completed }) }, completed: function (todos) { return todos.filter(function (todo) { return todo.completed }) } } // 创建Vue的实例(instance) var app = Vue.createApp({ // 设置页面用到的各种变量 data() { return { todos: todoStorage.fetch(), newTodo: '', editedTodo: null, visibility: 'all' } }, // 监听变量的修改 watch: { todos: { handler: function (todos) { todoStorage.save(todos) }, deep: true } }, // 定义computed properties computed: { filteredTodos: function () { return filters[this.visibility](this.todos) }, remaining: function () { return filters.active(this.todos).length }, allDone: { get: function () { return this.remaining === 0 }, set: function (value) { this.todos.forEach(function (todo) { todo.completed = value }) } } }, // 定义各种方法 // 实现对于待办事项的增加、删除和修改 // 注意,这里没有任何直接操作HTML DOM的代码 methods: { // 新增待办事项 addTodo: function () { var value = this.newTodo && this.newTodo.trim() if (!value) { return } this.todos.push({ id: todoStorage.uid++, title: value, completed: false }) this.newTodo = '' }, // 删除待办事项 removeTodo: function (todo) { this.todos.splice(this.todos.indexOf(todo), 1) }, // 编辑待办事项 editTodo: function (todo) { this.beforeEditCache = todo.title this.editedTodo = todo }, // 把待办事项标记为:已完成 doneEdit: function (todo) { if (!this.editedTodo) { return } this.editedTodo = null todo.title = todo.title.trim() if (!todo.title) { this.removeTodo(todo) } }, // 取消编辑 cancelEdit: function (todo) { this.editedTodo = null todo.title = this.beforeEditCache }, }, // 使用了自定义的Directive,也就是HTML中的<todo-focus> directives: { 'todo-focus': function (el, binding) { if (binding.value) { el.focus() } } } }) // 最后,使用Vue.js 渲染整个页面 app.mount('.todoapp') } </script>
该例子总共178行,代码精炼、功能齐备。可以看出,使用Vue.js做开发的效率非常高。
但是,一旦项目的需求增加,代码也会越来越膨胀,把HTML、JavaScript和CSS代码都写在一个文件中并不合适,所以需要以一种更好的形式来组织文件,这就是Webpack框架下的Vue.js。