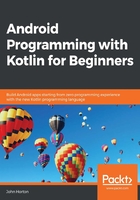
Some other overridden functions
You may have noticed that there are two other autogenerated functions in the code of all our projects using the Basic Activity template. They are onCreateOptionsMenu
and onOptionsItemSelected
. Many Android apps have a pop-up menu, so Android Studio generates one by default when using the Basic Activity template, including the outline of the code to make it work.
You can see the XML that describes the menu in res/menu/menu_main.xml
from the project explorer. The key lines of XML code are as follows:
<item android:id="@+id/action_settings" android:orderInCategory="100" android:title="@string/action_settings" app:showAsAction="never" />
This describes a menu item with the Settings text. If you run any of our apps built with the Basic Activity template, you will see the button as shown in the following screenshot:
If you tap the button, you can see it in action as follows:
So, how do the onCreateOptionsMenu
and onOptionsItemSelected
functions produce these results?
The onCreateOptionsMenu
function loads the menu from the menu_main.xml
file with this line of code:
menuInflater.inflate(R.menu.menu_main, menu)
It is called by the default version of the onCreate
function, which is why we don't see it happen.
Note
We will use the pop-up menu in Chapter 17, Data Persistence and Sharing, to switch between the different screens of our app.
The onOptionsItemSelected
function is called when the user taps on the menu button. This function handles what will happen when an item is selected. Now, nothing happens; it just returns true
.
Feel free to add Toast
and Log
messages to these functions to test out the order and timing I have just described.
Now that we have seen how the Android lifecycle works and have been introduced to a number of overridable functions to interact with this lifecycle, we had better learn the fundamentals of Kotlin so that we can write some more useful code to go in these functions and write our own functions too.