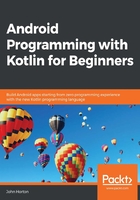
The structure of Kotlin code – revisited
We have already seen that each time we create a new Android project, we also create a new package; this is a kind of container for the code that we write.
We have also learned about and played around with classes. We have imported and taken direct advantage of classes from the Android API, such as Log
and Toast
. We have also used the AppCompatActivity
class but in a different manner to that of Log
and Toast
. You might recall that the first line of code in all our projects so far, after the import
statements, used the :
notation to inherit from a class:
class MainActivity : AppCompatActivity() {
When we inherit from a class, as opposed to just importing it, we are making it our own. In fact, if you take another look at the line of code, you can see that we are making a new class, with a new name, MainActivity
, but basing it on the AppCompatActivity
class from the Android API.
Note
AppCompatActivity
is a modified version of Activity
. It gives extra features for older versions of Android that would otherwise not be present. Everything we have discussed about Activity
, such as the lifecycle, is equally true for AppCompatActivity
. If the name ends in ...Activity
, it doesn't matter because everything we have discussed and will discuss is equally true. I will usually just refer to this class simply as Activity
.
We can summarize our use of classes as follows:
- We can import classes to use them
- We can inherit from classes to use them and to extend their functionality
- We can eventually make our own classes (and will do so soon)
Our own classes, and those that are written by others, are the building blocks of our code, and the functions within the classes wrap the functional code – that is, the code that does the work.
We can write functions within the classes that we extend as we did with topClick
and bottomClick
in Chapter 2, Kotlin, XML, and the UI Designer. Furthermore, we overrode functions that are already part of classes written by others, such as onCreate
and onPause
.
The only code, however, that we put in these functions was a few calls using Toast
and Log
. Now we are ready to take some more steps with Kotlin.