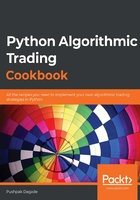
Creating timedelta objects
The datetime module provides a timedelta class, which can be used to represent information related to date and time differences. In this recipe, you will create timedelta objects and perform operations on them.
How to do it…
Follow along with these steps to execute this recipe:
- Import the necessary module from the Python standard library:
>>> from datetime import timedelta
- Create a timedelta object with a duration of 5 days. Assign it to td1 and print it:
>>> td1 = timedelta(days=5)
>>> print(f'Time difference: {td1}')
We get the following output:
Time difference: 5 days, 0:00:00
- Create a timedelta object with a duration of 4 days. Assign it to td2 and print it:
>>> td2 = timedelta(days=4)
>>> print(f'Time difference: {td2}')
We get the following output:
Time difference: 4 days, 0:00:00
- Add td1 and td2 and print the output:
>>> print(f'Addition: {td1} + {td2} = {td1 + td2}')
We get the following output:
Addition: 5 days, 0:00:00 + 4 days, 0:00:00 = 9 days, 0:00:00
- Subtract td2 from td1 and print the output:
>>> print(f'Subtraction: {td1} - {td2} = {td1 - td2}')
We will get the following output:
Subtraction: 5 days, 0:00:00 - 4 days, 0:00:00 = 1 day, 0:00:00
- Multiply td1 with a number (a float) :
>>> print(f'Multiplication: {td1} * 2.5 = {td1 * 2.5}')
We get the following output:
Multiplication: 5 days, 0:00:00 * 2.5 = 12 days, 12:00:00
How it works...
In step 1, you import the timedelta class from the datetime module. In step 2 you create a timedelta object that holds a time difference value of 5 days and assign it to td1. You call the constructor to create the object with a single attribute, days. You pass the value as 5 here. Similarly, in step 3, you create another timedelta object, which holds a time difference value of 4 days and assign it to td2.
In the next steps, you perform operations on the timedelta objects. In step 4, you add td1 and td2. This returns another timedelta object which holds a time difference value of 9 days, which is the sum of the time difference values held by td1 and td2. In step 5, you subtract td2 from td1. This returns another timedelta object that holds a time difference value of 1 day, which is the difference of time difference values held by td1 and td2. In step 6, you multiply td1 with 2.5, a float. This again returns a timedelta object that holds a time difference value of twelve and a half days.
There's more
A timedelta object can be created using one or more optional arguments:

In step 2 and step 3, we have used just the days argument. You can use other arguments as well. Also, these attributes are normalized upon creation. This normalization of timedelta objects is done to ensure that there is always a unique representation for every time difference value which can be held. The following code demonstrates this:
- Create a timedelta object with hours as 23, minutes as 59, and seconds as 60. Assign it to td3 and print it. It will be normalized to a timedelta object with days as 1 (and other date and time-related attributes as 0):
>>> td3 = timedelta(hours=23, minutes=59, seconds=60)
>>> print(f'Time difference: {td3}')
We get the following output:
Time difference: 1 day, 0:00:00
The timedelta objects have a convenience method, total_seconds(). This method returns a float which represents the total seconds contained in the duration held by the timedelta object.
- Call the total_seconds() method on td3. You get 86400.0 as the output:
>>> print(f'Total seconds in 1 day: {td3.total_seconds()}')
We get the following output:
Total seconds in 1 day: 86400.0