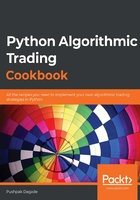
Creating datetime objects
The datetime module provides a datetime class, which can be used to accurately capture information relating to timestamps, dates, times, and time zones. In this recipe, you will create datetime objects in multiple ways and introspect their attributes.
How to do it…
Follow these steps to execute this recipe:
- Import the necessary module from the Python standard library:
>>> from datetime import datetime
- Create a datetime object holding the current timestamp using the now() method and print it:
>>> dt1 = datetime.now()
>>> print(f'Approach #1: {dt1}')
We get the following output. Your output will differ:
Approach #1: 2020-08-12 20:55:39.680195
- Print the attributes of dt1 related to date and time:
>>> print(f'Year: {dt1.year}')
>>> print(f'Month: {dt1.month}')
>>> print(f'Day: {dt1.day}')
>>> print(f'Hours: {dt1.hour}')
>>> print(f'Minutes: {dt1.minute}')
>>> print(f'Seconds: {dt1.second}')
>>> print(f'Microseconds: {dt1.microsecond}')
>>> print(f'Timezone: {dt1.tzinfo}')
We get the following output. Your output would differ:
Year: 2020
Month: 8
Day: 12
Hours: 20
Minutes: 55
Seconds: 39
Microseconds: 680195
Timezone: None
- Create a datetime object holding the timestamp for 1st January 2021::
>>> dt2 = datetime(year=2021, month=1, day=1)
>>> print(f'Approach #2: {dt2}')
You will get the following output:
Approach #2: 2021-01-01 00:00:00
- Print the various attributes of dt2 related to date and time:
>>> print(f'Year: {dt.year}')
>>> print(f'Month: {dt.month}')
>>> print(f'Day: {dt.day}')
>>> print(f'Hours: {dt.hour}')
>>> print(f'Minutes: {dt.minute}')
>>> print(f'Seconds: {dt.second}')
>>> print(f'Microseconds: {dt.microsecond}')
>>> print(f'Timezone: {dt2.tzinfo}')
You will get the following output:
Year: 2021
Month: 1
Day: 1
Hours: 0
Minutes: 0
Seconds: 0
Microseconds: 0
Timezone: None
How it works...
In step 1, you import the datetime class from the datetime module. In step 2, you create and print a datetime object using the now() method and assign it to dt1. This object holds the current timestamp information.
A datetime object has the following attributes related to date, time, and time zone information:

In step 3, these attributes are printed for dt1. You can see that they hold the current timestamp information.
In step 4, you create and print another datetime object. This time you create a specific timestamp, which is 1st Jan 2021, midnight. You call the constructor itself with the parameters—year as 2021, month as 1, and day as 1. The other time related attributes default to 0 and time zone defaults to None. In step 5, you print the attributes of dt2. You can see that they hold exactly the same values as you had passed to the constructor in step 4.
There's more
You can use the date() and time() methods of the datetime objects to extract the date and time information, as instances of datetime.date and datetime.time classes respectively:
- Use date() method to extract date from dt1. Note the type of the return value.
>>> print(f"Date: {dt1.date()}")
>>> print(f"Type: {type(dt1.date())}")
You will get the following output. Your output may differ::
Date: 2020-08-12
Type: <class 'datetime.date'>
- Use time() method to extract date from dt1. Note the type of the return value.
>>> print(f"Time: {dt1.time()}")
>>> print(f"Type: {type(dt1.time())}")
We get the following output. Your output may differ:
Time: 20:55:39.680195
Type: <class 'datetime.time'>
- Use date() method to extract date from dt2. Note the type of the return value.
>>> print(f"Date: {dt2.date()}")
>>> print(f"Type: {type(dt2.date())}")
We get the following output:
Date: 2021-01-01
Type: <class 'datetime.date'>
- Use time() method to extract date from dt2. Note the type of the return value.
>>> print(f"Time: {dt2.time()}")
>>> print(f"Type: {type(dt2.time())}")
We get the following output:
Time: 00:00:00
Type: <class 'datetime.time'>