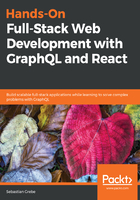
Connecting to a database with Sequelize
The first step is to initialize the connection from Sequelize to our MySQL server. To do this, we will create a new folder and file, as follows:
mkdir src/server/database
touch src/server/database/index.js
Inside of the index.js database, we will establish a connection to our database with Sequelize. Internally, Sequelize relies on the mysql2 package, but we do not use it on our own, which is very convenient:
import Sequelize from 'sequelize';
const sequelize = new Sequelize('graphbook_dev', 'devuser', 'PASSWORD', {
host: 'localhost',
dialect: 'mysql',
operatorsAliases: false,
pool: {
max: 5,
min: 0,
acquire: 30000,
idle: 10000,
},
});
export default sequelize;
As you can see, we require Sequelize from the node_modules, and then create an instance of it. The following properties are important for Sequelize:
- We pass the database name as the first parameter, which we just created.
- The second and third parameters are the credentials of our devuser. Replace them with the username and password that you entered for your database. The devuser has all user rights, and can read and write all of the databases in our MySQL server. This makes development a lot easier.
- The fourth parameter is a general options object that can hold many more properties. The preceding object is an example configuration.
- The host of our MySQL database is our local machine alias, localhost. If this is not the case, you can also specify the IP or URL of the MySQL server.
- The dialect is, of course, mysql.
- The operatorsAliases property specifies which strings can be used as aliases by Sequelize, or whether they can be used at all. An example would look as follows:
[Op.gt]: 6 // > 6
$gt: 6 // same as using Op.gt (> 6)
This example is taken from the Sequelize documentation. Generally, it is discouraged to use operators aliases at all. This is why you should disable it, and should always sanitize user input, to avoid SQL injections.
- With the pool option, you tell Sequelize the configuration for every database connection. The preceding configuration allows for a minimum of zero connections, which means that Sequelize should not maintain one connection, but should create a new one whenever it is needed. The maximum number of connections is five. This option also relates to the number of replica sets that your database system has.
- The idle field of the pool option specifies how long a connection can be unused before it gets closed and removed from the pool of active connections.
- When trying to establish a new connection to our MySQL server, the timeout before the connection is aborted is defined by the acquire option. In cases in which a connection cannot be created, this option helps to stop your server from freezing.
Executing the preceding code will instantiate Sequelize, and will successfully create a connection to our MySQL server. Going further, we need to handle multiple databases for every environment in which our application can run, from development to production. You will see that in the next section.