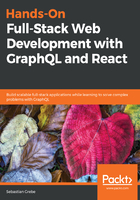
Using a configuration file with Sequelize
The previous setup for our database connection with Sequelize is fine, but it is not made for later deployment. The best option is to have a separate configuration file that is read and used according to the environment that the server is running in.
For this, create a new index.js file inside a separate folder (called config), next to the database folder:
mkdir src/server/config
touch src/server/config/index.js
Your sample configuration should look like the following code, if you have followed the instructions for creating a MySQL database. The only thing that we did here was to copy our current configuration into a new object indexed with the development or production environment:
module.exports = {
"development": {
"username": "devuser",
"password": "PASSWORD",
"database": "graphbook_dev",
"host": "localhost",
"dialect": "mysql",
"operatorsAliases": false,
"pool": {
"max": 5,
"min": 0,
"acquire": 30000,
"idle": 10000
}
},
"production": {
"host": process.env.host,
"username": process.env.username,
"password": process.env.password,
"database": process.env.database,
"logging": false,
"dialect": "mysql",
"operatorsAliases": false,
"pool": {
"max": 5,
"min": 0,
"acquire": 30000,
"idle": 10000
}
}
}
Sequelize expects a config.json file inside of this folder by default, but this setup will allow us a more custom approach in later chapters. The development environment directly store the credentials for your database whereas the production configuration uses environment variables to fill them.
We can remove the configuration that we hardcoded earlier and replace the contents of our index.js database file to require our configFile, instead.
This should look like the following code snippet:
import Sequelize from 'sequelize';
import configFile from '../config/';
const env = process.env.NODE_ENV || 'development';
const config = configFile[env];
const sequelize = new Sequelize(config.database, config.username,
config.password, config);
const db = {
sequelize,
};
export default db;
In the preceding code, we are using the NODE_ENV environmental variable to get the environment that the server is running in. We read the config file and pass the correct configuration to the Sequelize instance. The environmental variable will allow us to add a new environment, such as production, at a later point in the book.
The Sequelize instance is then exported for use throughout our application. We use a special db object for this. You will see why we are doing this later on.
Next, you will learn how to generate and write models and migrations for all of the entities that our application will have.