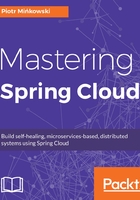
Enabling secure communication between client and server
Until now, none of the client's connections were being authenticated by the Eureka Server. While in the development mode, security doesn't really matter as much as in the production mode. The lack of it may be a problem. We would like to have, as a bare minimum, the discovery server secured with basic authentication to prevent unauthorized access to any service that knows its network address. Although Spring Cloud reference material claims that HTTP basic authentication will be automatically added to your Eureka Client, I had to include a starter with security to the project dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Then, we should enable security and set the default credentials by changing the configuration settings in the application.yml file:
security:
basic:
enabled: true
user:
name: admin
password: admin123
Now, all HTTP API endpoints and the Eureka dashboard are secured. To enable the basic authentication mode on the client side, the credentials should be provided within the URL connection address, as you can see in the following configuration settings. An example application that implements secure discovery is available in the same repository (https://github.com/piomin/sample-spring-cloud-netflix.git) as the basic example, but you need to switch to the security branch (https://github.com/piomin/sample-spring-cloud-netflix/tree/security). Here's the configuration that enabled HTTP basic authentication on the client side:
eureka:
client:
serviceUrl:
defaultZone: http://admin:admin123@localhost:8761/eureka/
For more advanced use, such as secure SSL connection with certificate authentication between discovery client and server, we should provide a custom implementation of DiscoveryClientOptionalArgs. We will discuss such an example in Chapter 12, Securing an API, specifically dedicated to security for Spring Cloud applications.