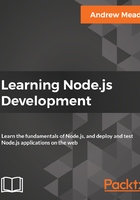
Printing a message of removing notes
Now, the next thing we'll do is print a message depending on whether or not a note was actually removed. That means app.js, which calls the removeNote function, will need to know whether or not a note was removed. And how do we figure that out? How can we possibly return that given the information we have in notes.js removeNotes function?
Well, we can, because we have two really important pieces of information. We have the length of the original notes array and we have the length of the new notes array. If they're equal then we can assume that no note was removed. If they are not equal, we'll assume that a note was removed. And that is exactly what we'll do.
If the removeNote function returns true, that means a note was removed; if it returns false, that means a note was not removed. In the removeNotes function we can add return, as shown in the following code. We'll check if notes.length does not equal filteredNotes.length:
var removeNote = (title) => {
var notes = fetchNotes();
var filteredNotes = notes.filter((note) => note.title !== title);
saveNotes(filteredNotes);
return notes.length !== filteredNotes.length;
};
If they're not equal it will return true, which is what we want because a note was removed. If they're equal it will return false, which is great.
Now, inside of app.js we can add a few lines in the removeNote, else if block to make the output for this command a little nicer. The first thing to do is to store that Boolean. I'll make a variable called noteRemoved and we'll set that equal to the return, result as shown in the following code, which will either be true or false:
} else if (command == 'remove') {
var noteRemoved = notes.removeNote(argv.title);
}
On the next line, we can create our message, and I'll do this all on one line using the ternary operator. Now, the ternary operator lets you specify a condition. In our case, we'll use a var message and it will be set equal to the condition noteRemoved, which will be true if a note was removed and false if it wasn't.
After the condition, we'll put a space with a question mark and a space; this is the statement that will run if it's true. If the noteRemoved condition passes, what we want to do is set message equal to Note was removed:
var message = noteRemoved ? 'Note was removed' :
Now, if noteRemoved is false, we can specify that condition right after the colon in the previous statement. Here, if there is no note removed we'll use the text Note not found:
var message = noteRemoved ? 'Note was removed' : 'Note not found';
Now with this in place, we can test out our message. The last thing to do is print the message to the screen using console.log passing in message:
var noteRemoved = notes.removeNote(argv.title);
var message = noteRemoved ? 'Note was removed' : 'Note not found';
console.log(message);
This lets us avoid if statements that make our else-if clause to remove unnecessarily complex.
Back inside of Atom we can rerun the last command, and in this case no note will get removed because we already deleted it. And when I run it, you can see that Note not found prints to the screen:

Now I'll remove a note that does exist; in notes-data.json I have a note with a title of secret as shown here:

Let's rerun the command removing the 2 from the title in Terminal. When I run this command, you can see Note was removed prints to the screen:

That is it for this section; we now have our remove command in place.