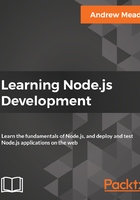
Reading note
In this section, you will be responsible for filling out the rest of the read command. Now, the read command does have an else-if block to find in app.js where we call getNote:
} else if (command === 'read') {
notes.getNote(argv.title);
getNote is defined over inside notes.js, even though currently it just prints out some dummy text:
var getNote = (title) => {
console.log('Getting note', title);
};
What you'll need to do in this section is wire up both of these functions.
First up, you will need to do something with the return value from getNote. Our getNote function will return the note object if it finds it. If it doesn't, it will return undefined just like we do for addNote discussed in the section Adding and saving note, in the previous chapter.
After you store that value, you'll do some printing using console.log, similar to what we have here:
if (command === 'add') {
var note = notes.addNote(argv.title, argv.body);
if (note) {
console.log('Note created');
console.log('--');
console.log(`Title: ${note.title}`);
console.log(`Body: ${note.body}`);
} else {
console.log('Note title taken');
}
Obviously, Note created will be something like Note read and Note title taken will be something like Note not found, but the general flow is going to be exactly the same. Now, once you have that wired up inside of app.js, you can move on to notes.js, filling out the function.
Now, the function inside of notes.js isn't going to be that complex. All you need to do is fetch the notes, like we've done in previous methods, then you're going to use notes.filter, which we explored to only return notes whose title matches the title passed in as the argument. Now, in our case this is either going to be zero notes, which means the note is not found, or it's going to be one note, which means we've found the note that the person wants to return.
Next, we do need to return that note. It's important to remember the return value from notes.filter is always going to be an array, even if that array only has one item. What you're going to need to do is return the first item in the array. If that item doesn't exist that's fine, it'll return undefined, as we want. If it does exist, great, that means we found the note. This method only requires three lines of code, one for fetching, one for filtering, and the return statement. Now, once you have all that done we'll test it out.