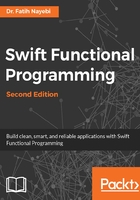
Defining and using function parameters
In function definition, parameters follow the function name and they are constants by default so we will not be able to alter them inside the function body.
Parameters should be inside parentheses. If we do not have any parameters, we simply put open and close parentheses without any characters between them as follows:
func functionName() { }
In FP, it is important to have functions that have at least one parameter. Remember, a function in mathematics is a relation between a set of inputs and outputs. So, we always have at least an input in a function and the same principle applies to FP.
As you'll already know or have guessed, we can have multiple parameters in function definition. Parameters are separated by commas and are named, so we need to provide the parameter name and type after a colon (:), as shown in the following example:
func functionName(param1: ParamType, param2: ParamType) { }
// To call:
functionName(param1: parameter, param2: secondParam)
ParamType, which is an example type, can also be an optional type so the function becomes the following if our parameters need to be optionals:
func functionName(param1: ParamType?, param2: ParamType?) { }
Swift enables us to provide external parameter names that will be used when functions are called. The following example presents the syntax:
func functionName(externalParamName localParamName: ParamType)
// To call:
functionName(externalParamName: parameter)
Only a local parameter name is usable in the function body.
It is possible to omit parameter names with the _ syntax; for instance, if we do not want to provide any parameter name when the function is called, we can use _ as the externalParamName for the second or subsequent parameters.
If we want to have a parameter name for the first parameter name in function calls, we can basically provide the local parameter name as external also. In this book, we are going to use the default function parameter definition.
Parameters can have default values as follows:
func functionName(param: Int = 3) {
print("\(param) is provided.")
}
functionName(5) // prints "5 is provided."
functionName() // prints "3 is provided"
Parameters can be defined as inout to enable function callers obtaining parameters that are going to be changed in the body of a function. As we can use tuples for function returns, it is not recommended to use inout parameters unless we really need them.
We can define function parameters as tuples. For instance, the following example function accepts a tuple of the (Int, Int) type:
func functionWithTupleParam(tupleParam: (Int, Int)) {}
In Swift, parameters can be of a generic type. The following example presents a function that has two generic parameters. In this syntax, any type (for example, T or V) that we put inside <> should be used in parameter definition:
func functionWithGenerics<T, V>(firstParam: T, secondParam: V)
We will cover generics in Chapter 5, Generics and Associated Type Protocols; at this point, knowing the syntax should be enough.
We are almost over with function parameters, finally! Last but not least, we are going to look into variadic functions in the next section.