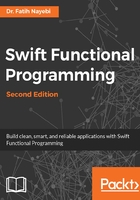
Defining and using variadic functions
Swift enables us to define functions with variadic parameters. A variadic parameter accepts zero or more values of a specified type. Variadic parameters are similar to array parameters but they are more readable and can only be used as the last parameter in multi-parameter functions.
As variadic parameters can accept zero values, we will need to check whether they are empty.
The following example presents a function with variadic parameters of the String type:
func greet(names: String...) {
for name in names {
print("Greetings, \(name)")
}
}
// To call this function
greet(names: "Josee", "Jorge") // prints twice
greet(names: "Josee ", "Jorge ", "Marcio") // prints three times
The most boring part of the chapter is almost over. Seriously, we needed to master the Swift function/method syntax and we will see the benefits in upcoming chapters. If you speed read or skipped previous sections, it is okay. The upcoming sections are going to be essential for FP so they need more attention.