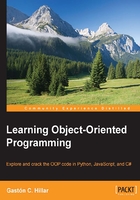
Declaring classes in Python
Throughout this book, we will work with Python 3.4.3. However, all the explanations and code samples are compatible with Python 3.x.x. Therefore, you can work with previous Python versions as long as the major version number is 3. We will use JetBrains PyCharm Community Edition 4 as the main Python IDE and the supplier of an interactive Python console. However, you can use your favorite Python IDE or just the Python console.
Note
Everything is a class in Python, that is, all the elements that can be named in Python are classes. Guido van Rossum designed Python according to the first-class everything principle. Thus, all the types are classes, from the simplest types to the most complex ones: integers, strings, lists, dictionaries, and so on. This way, there is no difference between an integer (int
), a string
, and a list
. Everything is treated in the same way. Even functions, methods, and modules are classes.
For example, when we enter the following lines in a Python console, we create a new instance of the int
class. The console will display <class 'int'>
as a result of the second line. This way, we know that area
is an instance of the int
class:
area = 250 type(area)
When we type the following lines in a Python console, we create a new instance of the function
class. The console will display <class 'function'>
as a result of the second line. Thus, calculateArea
is an instance of the function
class:
def calculateArea(width, height): return width * height type(CalculateArea)
Let's analyze the simple calculateArea
function. This function receives two arguments: width and height. It returns the width value multiplied by the height value. If we call the function with two int
values, that is, two int
instances, the function will return a new instance of int
with the result of width
multiplied by height
. The following lines call the calculateArea
function and save the returned int
instance in the rectangleArea
variable. The console will display <class 'int'>
as a result of the third line. Thus, rectangleArea
is an instance of the int
class:
rectangleArea = calculateArea(300, 200) print(rectangleArea) type(rectangleArea)
The following lines create a new minimal Rectangle
class in Python:
class Rectangle: pass
The class
keyword followed by the class name (Rectangle
) and a colon (:
) composes the header of the class definition. In this case, the class doesn't have a parent class or a superclass. Therefore, there aren't superclasses enclosed in parentheses after the class name and before the colon (:
). The indented block of statements that follows the class definition composes the body of the class. In this case, there is just a single statement, pass
, and the class doesn't define either attributes or methods. The Rectangle
class is the simplest possible class we can declare in Python.
Tip
Any new class you create that doesn't specify a superclass will be a subclass of the builtins.object
class. Thus, the Rectangle
class is a subclass of builtins.object
.
The following line prints True
as a result in a Python console, because the Rectangle
class is a subclass of object
:
issubclass(Rectangle, object)
The following lines represent an equivalent way of creating the Rectangle
class in Python. However, we don't need to specify that the class inherits from an object because it adds unnecessary boilerplate code:
class Rectangle(object): pass