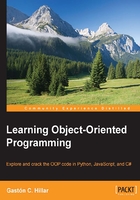
Customizing constructors in Python
We want to initialize instances of the Rectangle
class with the values of both width
and height
. After we create an instance of a class, Python automatically calls the __init__
method. Thus, we can use this method to receive both the width
and height
arguments. We can then use these arguments to initialize attributes with the same names. We can think of the __init__
method as the equivalent of a constructor in other object-oriented programming languages.
The following lines create a Rectangle
class and declare an __init__
method within the body of the class:
class Rectangle: def __init__(self, width, height): print("I'm initializing a new Rectangle instance.") self.width = width self.height = height
This method receives three arguments: self
, width
, and height
. The first argument is a reference to the instance that called the method. We used the name self
for this argument. It is important to notice that self
is not a Python keyword. It is just the name for the first argument, and it is usually called self
. The code within the method prints a message indicating that the code is initializing a new Rectangle
instance. This way, we will understand when the code within the __init__
method is executed.
Then, the following two lines create the width
and height
attributes for the instance and assign them the values received as arguments with the same names. We use self.width
and self.height
to create the attributes for the instance. We create two attributes for the Rectangle
instance right after its creation.
The following lines create two instances of the Rectangle
class named rectangle1
and rectangle2
. The Python console will display I'm initializing a new Rectangle instance.
after we enter each line in the Python console:
rectangle1 = Rectangle(293, 117) rectangle2 = Rectangle(293, 137)

The preceding screenshot shows the Python console. Each line that creates an instance specifies the class name followed by the desired values for both the width
and the height
as arguments enclosed in parentheses. If we take a look at the declaration of the __init__
method within the Rectangle
class, we will notice that we just need to specify the second and third arguments (width
and height
). Also, we just need to skip the required first parameter (self
). Python resolves many things under the hood. We just need to make sure that we specify the values for the required arguments after self
to successfully create and initialize an instance of Rectangle
.
After we execute the previous lines, we can check the values for rectangle1.width
, rectangle1.height
, rectangle2.width
, and rectangle2.height
.
The following line will generate a TypeError
error and won't create an instance of Rectangle
because we missed the two required arguments: width
and height
. The specific error message is TypeError: __init__() missing 2 required positional arguments: 'width' and 'height'
. The error message is shown in the following screenshot:
rectangleError = Rectangle()
