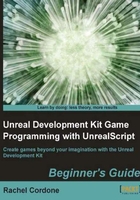
Time for action – Comparisons
- Let's take a look at two ints and the various comparison operators we can use on them.
var int Int1, Int2; function PostBeginPlay() { 'log(Int1 == Int2); } defaultproperties { Int1=5 Int2=5 }
Setting both of them to the same value and using the equal comparison gives us
True
in the log:[0007.79] ScriptLog: True
If the variables weren't exactly the same, we would get False.
- The opposite of this comparison is "Not Equal", which is denoted by an exclamation point followed by an equal sign. If we wanted to know if two variables weren't the same, we would use this.
var int Int1, Int2; function PostBeginPlay() { 'log(Int1 != Int2); } defaultproperties { Int1=3 Int2=5 }
Since they have different values, we'll get
True
in the log again:[0007.70] ScriptLog: True
Equal or not equal also apply to vectors and rotators. Each element in those structs is compared to each other, and it will return
False
if any of them are different. - For greater than or less than, we would simply use those symbols.
var int Int1, Int2; function PostBeginPlay() { 'log(Int1 < Int2); 'log(Int1 > Int2); } defaultproperties { Int1=3 Int2=5 }
And the log:
[0007.60] ScriptLog: True [0007.60] ScriptLog: False
- The same works for "greater than or equal to" and "less than or equal to", we simply follow it with an equal sign:
var int Int1, Int2; function PostBeginPlay() { 'log(Int1 <= Int2); 'log(Int1 >= Int2); } defaultproperties { Int1=5 Int2=5 }
The log for this:
[0007.45] ScriptLog: True [0007.45] ScriptLog: True
Greater than or less than do not apply to vectors or rotators and the compiler will give an error if we try to use them.
- A special comparison operator for floats and strings is the "approximately equal to" operator, denoted by a tilde followed by an equal sign (
~=
). For floats, it returns true, if they are within 0.0001 of each other, useful for making sure complicated equations don't have to return the exact same result, just close enough to account for rounding errors.var float Float1, Float2; function PostBeginPlay() { 'log(Float1 ~= Float2); } defaultproperties { Float1=1.0 Float2=1.000001 }
This returns
True
in the log:[0007.94] ScriptLog: True
- For strings, the "approximately equal to" operator is a case-insensitive comparison.
var string String1, String2; function PostBeginPlay() { 'log(String1 ~= String2); } defaultproperties { String1="STRING TEST" String2="string test" }
The log:
[0007.74] ScriptLog: True
As long as the letters are the same it will return true even if different letters are capitalized.
What just happened?
Comparisons, like arithmetic operators, are one of the basic things to know about a programming language. They'll be used all the time, and like arithmetic it's good to know how they interact with each variable type.
Logical operators
In logical operators, AND is expressed by two "and" signs (&&
), OR by two vertical bar or "pipe" characters (||
), and NOT is expressed using an exclamation point (!
). To understand logical operators, think about how we use those words in sentences. As an example, take a look at this sentence:
If it's not raining and we have enough money...
Expressing this in code with logical operators would look like this:
!bRaining && CurrentMoney > RequiredMoney
We can see the use of the NOT and AND logical operators. NOT raining AND current money > required money. Let's take a look at another example:
Tuesday or Thursday
In code that would look like this:
Day == "Tuesday" || Day == "Thursday"